Introduction
If you’re looking to create a set of shared resources that can be accessed by multiple applications, and you want to deploy and maintain a single copy of those resources, consider using a shared library.
Java classes that are deployed in a shared library are not available to the integration server-wide class loader. When you deploy a shared library that contains Java files, a new class loader is created for that shared library. This class loader contains all Java classes in the shared library, as well as the Java classes from all referenced shared libraries.
In this post, we will explore how to call Java static methods (In Compute Node) and java class (In Java Compute Node) from a shared library in two contexts: within a Compute Node using ESQL and in a Java Compute Node using Java classes. we will learn how to use shared libraries to centralize common Java functionality and access it efficiently across different integration scenarios.
Calling Java static methods from a Shared Library in Application’s Compute Node Using ESQL
Create a shared library with following structure and a Java class named “Utils.java” make sure to create a package.
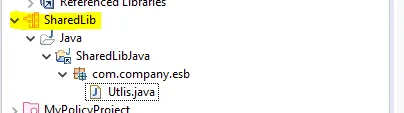
Add the following static method in “Utlis.java” class.
public static String sayHello(String sayHelloTo) {
return "Hello " + sayHelloTo;
}
Create an Application named “MyApp” and a message flow named “Flow1_esql.msgflow” in it. Drag and drop the following nodes.
HTTP Input: Select the input message parsing a JSON and Path suffix for URL as “/esql”.
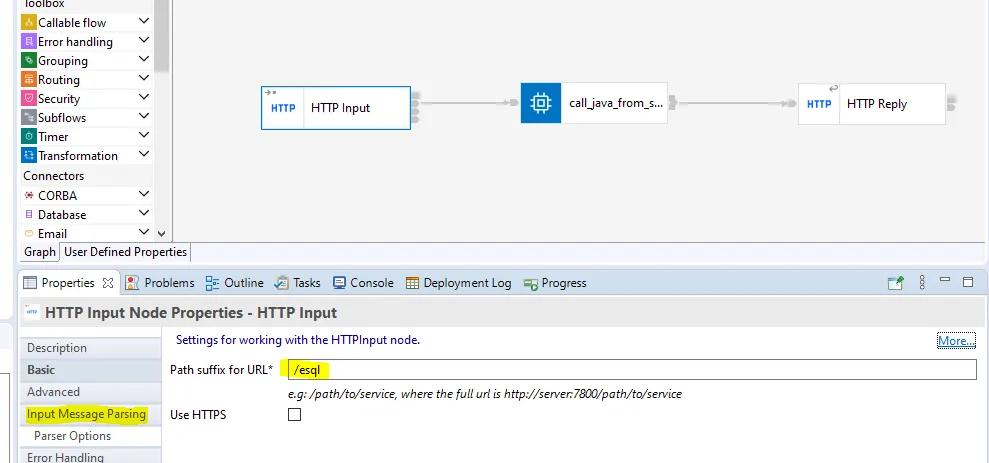
Compute Node: Double click the compute node and create a file named “Flow1_call_java_from_sharedLib.esql” in default namespace and write the following code in that file.
DECLARE sayHelloTo CHARACTER InputRoot.JSON.Data.sayHelloTo;
SET OutputRoot.JSON.Data.Response = sayHello(sayHelloTo);
And a function at the end of file. Notice the CLASSLOADER clause that is to access a Java classes in a referenced shared library called “SharedLib”
CREATE FUNCTION sayHello (IN sayHelloTo CHARACTER )
RETURNS CHARACTER
LANGUAGE JAVA
EXTERNAL NAME "com.company.esb.Utlis.sayHello"
CLASSLOADER "{SharedLib}";
HTTP Reply: To reply back to calling client.
Note: A shared library must be deployed with or before an application that references it. If you deploy resources by dragging them onto an integration server, deploy the shared library first. If you deploy resources by adding them to a BAR file, ensure that you include any shared libraries that your application references.
Now deploy the shared library first and then application by drag and drop on Integration Server or packaged it inside the application BAR file to deploy.
Send a message to flow as shown below, static Java method in shared library will concatenate the given name to “Hello”.
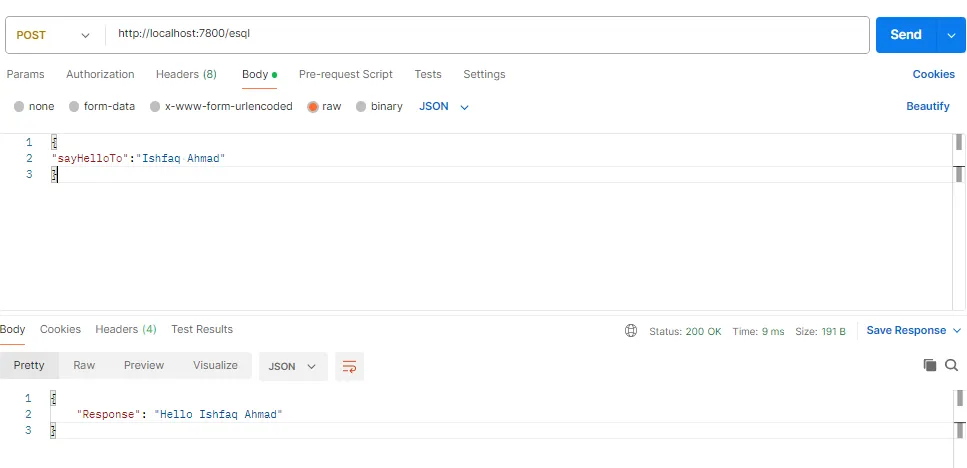
Calling Java class from a Shared Library in application’s Java Compute Node
Java classes in applications, static libraries, or independent projects cannot access Java classes or methods in shared libraries. but we can directly refer the java class on Java Compute Node. let’s see how.
Create another message flow named “Flow2_java.msgflow” and drag and drop the following nodes.
HTTP Input: Select the input message parsing as JSON and Path suffix for URL as “/java”.
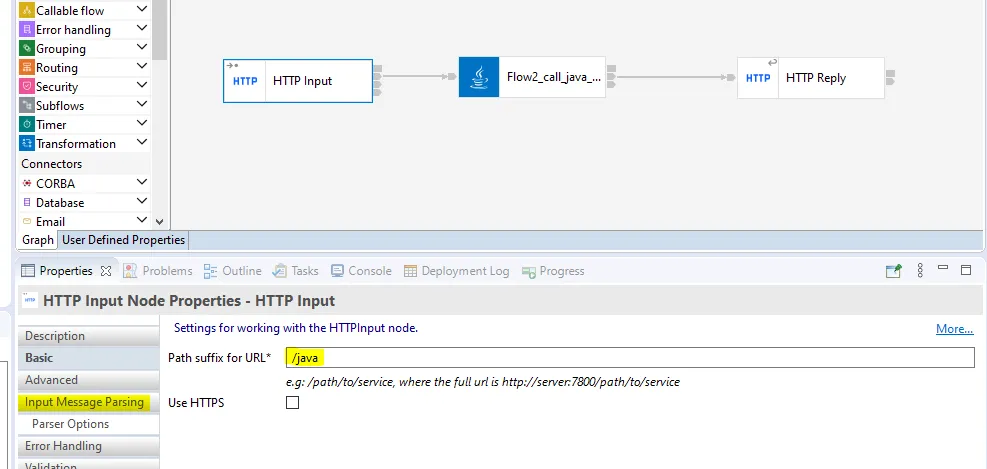
Java Compute Node: Double click on JCN select source folder as “SharedLibJava” and name file as “Flow2_call_javaclass_from_sharedLib”, and click finish.
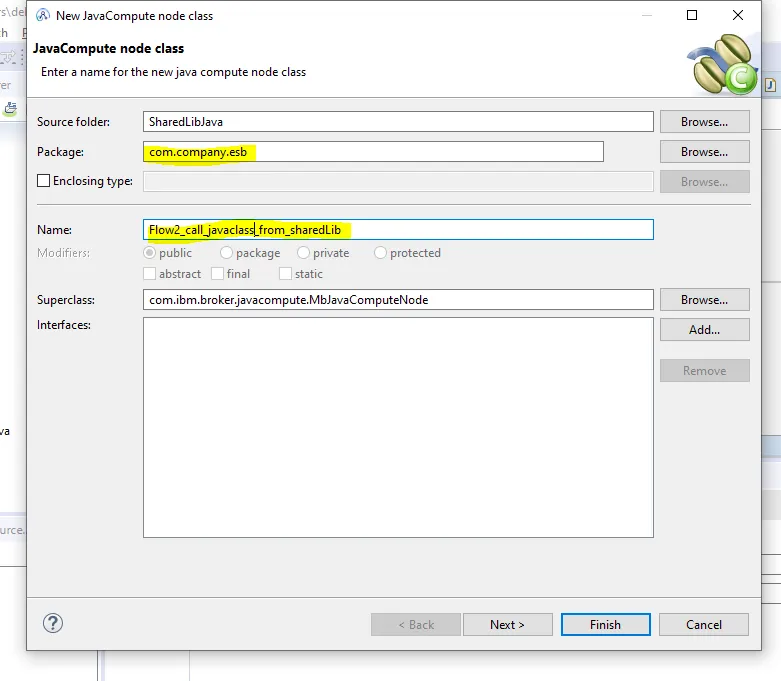
Click on JCN and type shared library name in “Java classloader service” as {SharedLib}.
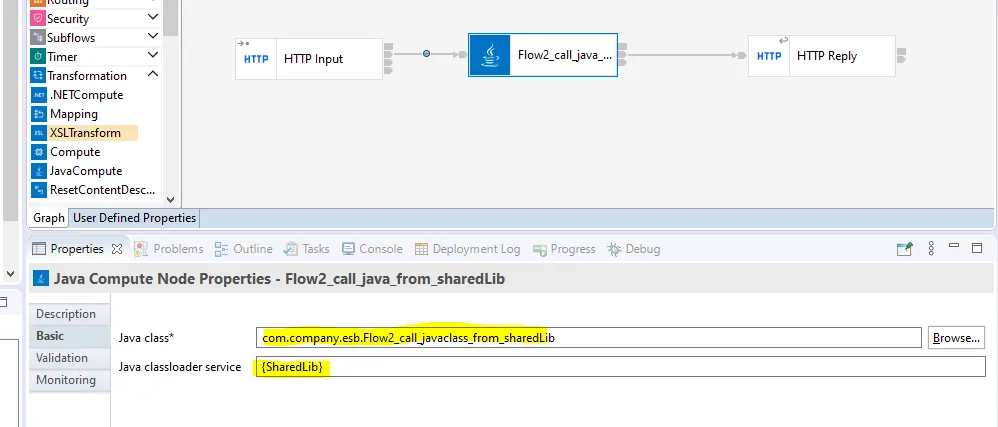
Add the following Java code in JCN.
MbElement Mbe = inAssembly.getMessage().getRootElement();
MbElement outRoot =
outMessage.getRootElement();
MbElement outJsonRoot =
outRoot.createElementAsLastChild(MbJSON.PARSER_NAME);
MbElement outJsonData =
outJsonRoot.createElementAsLastChild(MbElement.TYPE_NAME, MbJSON.DATA_ELEMENT_NAME, null);
outJsonData.createElementAsLastChild(MbElement.TYPE_NAME_VALUE, "Response",com.company.esb.Utlis.sayHello(Mbe.getFirstElementByPath("/JSON/Data/sayHelloTo").getValueAsString()));
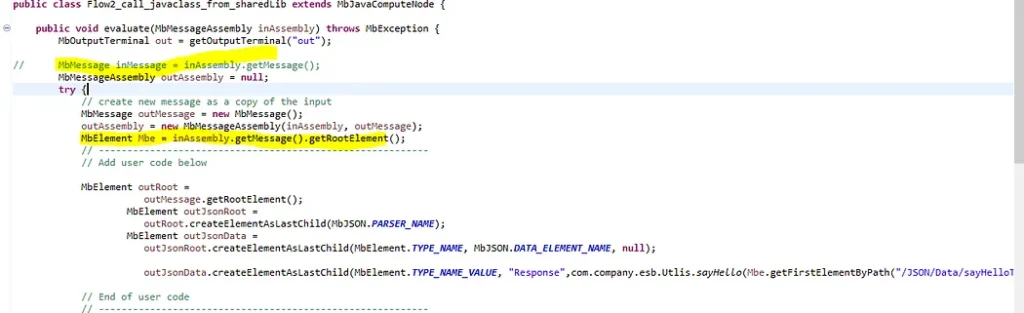
HTTP Reply: To reply back to calling client.
at this point shared library look like this.
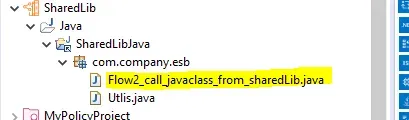
Final Application and shared lib structure looks like this.
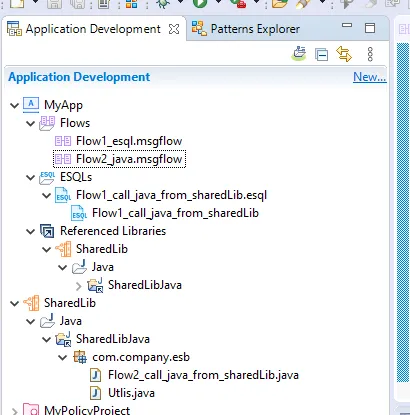
Now deploy shared lib and application on server, and test the java flow.
Send a message to flow as shown below, Java class from shared library that is refer on JCN will call the static method in “Utils.java” that concatenate the given name to “Hello”.
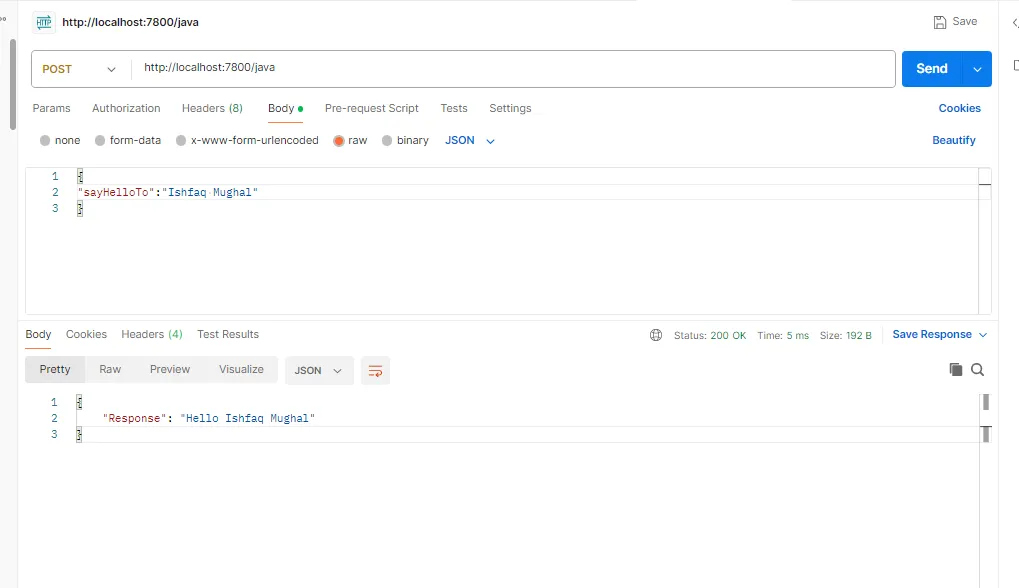
Conclusion!
In this post, we’ve covered how to call static Java methods from shared library in ESQL-based Compute Nodes and refer Java class in Java Compute Node from shared lib. Using shared libraries allows us to centralize common functionality and streamline your integration processes. By understanding how class loaders work and using the `CLASSLOADER` clause in ESQL, you can efficiently access static Java methods without duplicating code.
GitHub – ishfaq80/SharedLibProject
IBM App Connect Enterprise 11.0.0 – IBM Documentation
https://www.ibm.com/docs/en/app-connect/11.0.0?topic=libraries-shared-java-files
Leave a Reply